
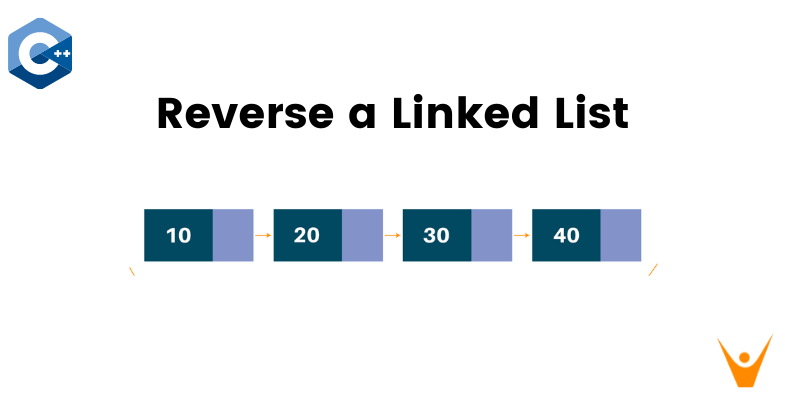
They can be used to implement stacks and queues, as well as other abstract data types. Linked lists are great for quickly adding and deleting nodes. Linked lists may be the second most used data structure, behind arrays. Head pointer: The memory address contained by the head node referencing the first node in the list.Tail: The last node in a linked list is called the tail.Next: The next node in a sequence, respective to a given list node.Node: The object in the list, with two components: a piece of data (element) and a pointer.Some terms associated with linked lists are: In the case of a singly linked list, the tail node’s pointer is null. A singly linked list is uni-directional while a doubly linked list is bi-directional. A node in a doubly linked list, on the other hand, stores two pointers: one to the next node, and one to the previous node in the sequence. In the case of a singly linked list, nodes only store one pointer to the next node in the sequence. Each node has two components: 1.) A data element and 2.) A pointer containing a memory address to the next node in the list. Upcoming next is another pattern on Linked List called the Slow & Fast Pointer approach.Linked lists are linear data structures connecting nodes in a sequence. It also provides you with a way of thinking and approaching any twisted Linked List question in your coding interviews. It is an important first step in your coding interview prep to make yourself comfortable handling pointers and references. While I know most companies don't test you on Linked List questions these days. LinkedList is a data structure which stores the data in a linear way. In this tutorial, we’ll be discussing the various algorithms to reverse a Linked List and then implement them using Java.

Returns an iterator over the elements in this deque in reverse sequential order. Reversing a Linked List is an interesting problem in data structure and algorithms.
Once you solve the above practice problems, you should be pretty comfortable with traversing around a singly linked list. public class LinkedListThe only tricky part is how you manage the pointers to perform the stitching of the reversed sublists.Ģ.2 Reverse Nodes in Alternate k-Groups - I didn't find this on Leetcode, but the only change in this problem is you reverse every alternate k-group of nodes.
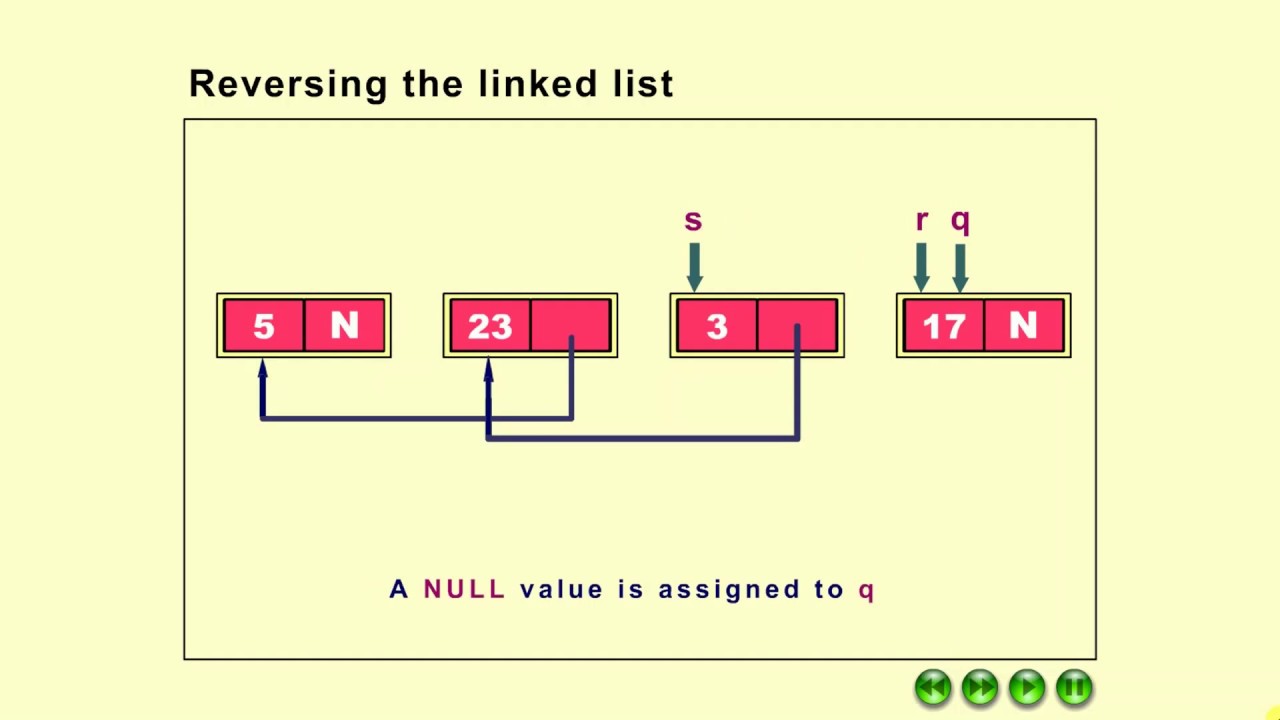

Reverse Nodes in k-Group - Conceptually it is the same as the problems we discussed in this post. Add Two Numbers II - Then look at this problem and think about how you can apply the reversal pattern hereĢ.1. Add Two Numbers - First practice this problemġ.2. You can apply the reversing of the linked list pattern to the following problems on Leetcode:ġ.1.
#Reverse a linked list code#
Here's a visual representation of the linked list reversal algorithm we discussed above: Visual flow for reversing a Singly Linked List Code: public Node reverse(ListNode head) Code to reverse a sub-list in Java Applying the Pattern. Because prev will now be pointing to the last node of the original list, and it is now the head of the newly reversed linked list. Keep iterating until curr reaches the end of the list and there are no more nodes to be reversed. So, now we can move the curr pointer to fwd, which is the new node we need to process for reversing.ģ.4. Because in step 3.1, we had already placed the fwd pointer ahead of curr. Given the head of a singly linked list, reverse the list, and return the reversed list. next) to the prev pointer.īasically, starting the reversal process for curr node.ģ.3. Make the curr node's next pointer ( curr. The only overhead of this algorithm is finding the end of the linked list. Place the fwd pointer on the node just after the Current Pointer ( curr).ģ.2. This way of reversing a linked list is pretty optimized and pretty fast. Initially, the prev and next pointers won't be referencing any nodeģ.1.Place the curr node at the head of the list.To reverse a linked list iteratively and in place, we need 3 new pointers: Previous (prev), Current (curr), and Forward (fwd) pointer. As the DLLNode maintains gives us two pointers, one pointing to the previous node and one pointing to the next node in the list. Our only way of traversing backward is to reverse the linked list.Ĭompared to Singly Linked List, traversing backward would have been easier if we had a Doubly Linked List. After moving, to go back to the previous node we won't have any reference information in our current Node data structure. This means we can only move forward from one node to the other as we always only maintain a pointer to only the next node in the list. We learned in the Basics of Linked Lists that traversal in a Singly Linked list is uni-directional.
